Arduboy ゲームプログラム 「ロボ」
Arduboyでゲーム開発へ戻る
●操作方法とルール
・左右ーで自機(ロボット)を左右に移動
・Aボタンでジャンプ、押し続けると滞空時間が伸びる
・Bボタンでレーザー発射、敵を自動でロックオンし破壊します
・敵にぶつかるとゲームオーバー
※より高い位置で敵を倒すとスコアが多く増えます
※本気でプレイするより、レーザーを発射し続けてストレスを解消するゲームです(笑)
・プログラムの行数 152行
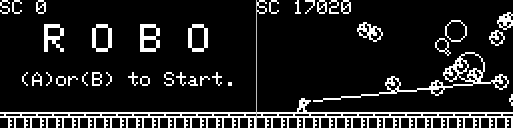
●ゲームサンプルコード04「ロボ」
#include <Arduboy2.h>
Arduboy2 arduboy;
BeepPin1 beep;
const unsigned char BMP[] PROGMEM = {
0x00, 0xc0, 0x7c, 0x3e, 0xf6, 0x9c, 0x0c, 0x04, // ロボ歩き
0x00, 0x00, 0x0e, 0xff, 0xeb, 0x8c, 0x0c, 0x04, // 〃
0x00, 0x00, 0xe0, 0x7e, 0x1f, 0x0b, 0x0c, 0x04, // ロボジャンプ
0x30, 0x18, 0xec, 0x7e, 0x1f, 0x0b, 0x0c, 0x04, // 〃
0x18, 0x6e, 0x4a, 0x9f, 0x99, 0x42, 0x66, 0x18, // ┬敵機(回転アニメ)
0x18, 0x66, 0x42, 0x99, 0x9f, 0x4a, 0x6e, 0x18, // │
0x18, 0x66, 0x42, 0x99, 0xf9, 0x52, 0x76, 0x18, // │
0x18, 0x76, 0x52, 0xf9, 0x99, 0x42, 0x66, 0x18, // ┘
0x07, 0x05, 0xfd, 0x55, 0x05, 0xfd, 0x05, 0x05 // 地面
};
char scene = 0;
int cntr = 0;
long int score = 0;
int pl_x, pl_y, pl_yp, pl_jmp; // プレイヤー(ロボ)キャラ用
const int EMAX = 50; // 敵 最大出現数
int ex[EMAX], ey[EMAX], ee[EMAX], emax;
void drawBmp(int x, int y, int n) { // ビットっマップ描画
arduboy.drawBitmap(x, y, BMP+n*8, 8, 8, WHITE);
}
void prt(int x, int y, char *t, int s) { // 文字列出力
arduboy.setCursor(x, y);
arduboy.setTextSize(s);
arduboy.print(t);
}
void setup() { // 初期化
arduboy.begin();
arduboy.setFrameRate(30);
beep.begin();
}
void loop() { // メインループ
int i;
if (!(arduboy.nextFrame())) return;
cntr++;
beep.timer();
arduboy.clear();
for(i=0; i<33; i++) drawBmp(i*8-(cntr/10)%8, 56, 8); // 地面
emax = 2 + score/50; // 敵出現数
if(emax>EMAX) emax = EMAX;
if(scene>1) { // 敵の処理
for(i=0; i<emax; i++) {
if(ee[i]>0) { // 爆発
if(ee[i]%2) arduboy.drawCircle(ex[i]+3, ey[i]+3, ee[i], WHITE);
ee[i]++;
if(ee[i]==16) {
ex[i] = 128+rand()%128;
ey[i] = rand()%48;
ee[i] = 0;
}
continue;
}
ex[i] = ex[i] - 1 - (i%3)/2;
if(ex[i]<-8) ex[i] = 128+rand()%128;
if(-8<ex[i] && ex[i]<128) {
drawBmp(ex[i], ey[i], 4+(cntr/2)%4);
if(scene==2) { // プレイヤーとのヒットチェック
int d = (ex[i]-pl_x)*(ex[i]-pl_x) + (ey[i]-pl_y)*(ey[i]-pl_y);
if(d<8*8) {
scene = 3;
cntr = 0;
}
}
}
}
}
switch(scene) {
case 0: // 初期化
pl_x = 30, pl_y = 48, pl_yp = 0, pl_jmp = 0;
for(i=0; i<EMAX; i++) {
ex[i] = 128+rand()%128;
ey[i] = rand()%40;
ee[i] = 0;
}
scene = 1;
break;
case 1: // タイトル
prt(22, 12, "R O B O", 2);
prt(10, 36, "(A)or(B) to Start.", 1);
if(arduboy.pressed(A_BUTTON) || arduboy.pressed(B_BUTTON)) {
scene = 2;
score = 0;
}
break;
case 2: // ゲーム
if(arduboy.pressed(LEFT_BUTTON) && pl_x>0) pl_x--;
if(arduboy.pressed(RIGHT_BUTTON) && pl_x<120) pl_x++;
if(pl_jmp==0) { // ジャンプ?
if(arduboy.pressed(A_BUTTON)) {
pl_yp = -4;
pl_jmp = 1;
}
}
else if(pl_jmp==1) { // ジャンプ中
pl_y += pl_yp;
if(arduboy.pressed(A_BUTTON))
pl_yp += (cntr%4)/3;
else
pl_yp += 1;
if(pl_y>=48) {
pl_y = 48;
pl_jmp = 2;
}
} // 着地後
else {
if(!arduboy.pressed(A_BUTTON)) pl_jmp = 0;
}
if(arduboy.pressed(B_BUTTON)) { // ビーム
for(i=0; i<emax; i++) {
if(ee[i]==0 && 0<ex[i] && ex[i]<128) {
ee[i] = 1;
score = score + 1 + (48-pl_y)/2;
arduboy.drawLine(pl_x+9, pl_y+2, ex[i]+3, ey[i]+3, WHITE);
beep.tone(beep.freq(800), 4);
break;
}
}
}
if(pl_jmp !=1)
drawBmp(pl_x, pl_y, (cntr/10)%2); // 歩行
else
drawBmp(pl_x, pl_y, 2+cntr%2); // ジャンプ姿勢
break;
case 3: // ゲームオーバー
if(cntr<30*4) {
for(i=0; i<4; i++) arduboy.drawCircle(pl_x+rand()%8, pl_y+rand()%8, 4, WHITE);
if(cntr%5==1) beep.tone(beep.freq(490-cntr*4), 2);
}
else if(cntr<30*8) {
prt(37, 24, "GAME OVER", 1);
}
else {
scene = 0;
}
break;
}
prt(0, 0, "SC ", 1);
arduboy.print(score*10);
arduboy.display();
}
Arduboyでゲーム開発のページへ移動